Introducing the Closure Library Editor
Wednesday, July 14, 2010 | 11:05 AM
One of the many UI widgets included in the Closure Library is a full-featured rich text editor.
All modern browsers have rich text editing functionality built in — unfortunately this functionality is at best inconsistent and, at worst, broken. Closure Library’s editor is a sub-project within Closure. It provides a common, powerful, and extensible interface that abstracts the cross-browser inconsistencies and shortcomings of rich text editing.
We use the Closure editor in Gmail, Google Sites, Google Groups, and many other Google products. We’re very happy to be able to share this editor with you as part of the Closure Library.
Using the editor in your project
A demo of the basic editor functionality is included in the source distribution, and can be tried here.
The most important code is as follows. First, we create an editable field and attach it to an element with the id
editMe
.// Create an editable field.
var myField = new goog.editor.Field('editMe');
Next, we register editing plugins. The rich text editor uses a plugin-based architecture in order to support many products with different feature sets. For example, in Gmail, the tab key moves the cursor to the send button, while in Google Sites it either adds 4 spaces or indents the current bulleted list. Plugins allow us to create modular features for the Editor and let each application pick and choose which ones they need or want. This ensures each application only brings in the code it needs, helping to minimize code size and latency.
// Create and register all of the editing plugins you want to use.
myField.registerPlugin(new goog.editor.plugins.BasicTextFormatter());
myField.registerPlugin(new goog.editor.plugins.RemoveFormatting());
myField.registerPlugin(new goog.editor.plugins.UndoRedo());
myField.registerPlugin(new goog.editor.plugins.ListTabHandler());
myField.registerPlugin(new goog.editor.plugins.SpacesTabHandler());
myField.registerPlugin(new goog.editor.plugins.EnterHandler());
myField.registerPlugin(new goog.editor.plugins.HeaderFormatter());
myField.registerPlugin(
new goog.editor.plugins.LoremIpsum('Click here to edit'));
myField.registerPlugin(
new goog.editor.plugins.LinkDialogPlugin());
In a future post, we’ll discuss how you can use the plugin system to add your own custom functionality to your editor.
Next we set up our toolbar:
// Specify the buttons to add to the toolbar, using built in default buttons.
var buttons = [
goog.editor.Command.BOLD,
goog.editor.Command.ITALIC,
goog.editor.Command.UNDERLINE,
goog.editor.Command.FONT_COLOR,
goog.editor.Command.BACKGROUND_COLOR,
goog.editor.Command.FONT_FACE,
goog.editor.Command.FONT_SIZE,
goog.editor.Command.LINK,
goog.editor.Command.UNDO,
goog.editor.Command.REDO,
goog.editor.Command.UNORDERED_LIST,
goog.editor.Command.ORDERED_LIST,
goog.editor.Command.INDENT,
goog.editor.Command.OUTDENT,
goog.editor.Command.JUSTIFY_LEFT,
goog.editor.Command.JUSTIFY_CENTER,
goog.editor.Command.JUSTIFY_RIGHT,
goog.editor.Command.SUBSCRIPT,
goog.editor.Command.SUPERSCRIPT,
goog.editor.Command.STRIKE_THROUGH,
goog.editor.Command.REMOVE_FORMAT
];
var myToolbar = goog.ui.editor.DefaultToolbar.makeToolbar(buttons,
goog.dom.getElement('toolbar'));
// Hook the toolbar into the field.
var myToolbarController =
new goog.ui.editor.ToolbarController(myField, myToolbar);
And finally, we turn on editing:
myField.makeEditable();
When we open the file in the browser, we get an HTML editor:
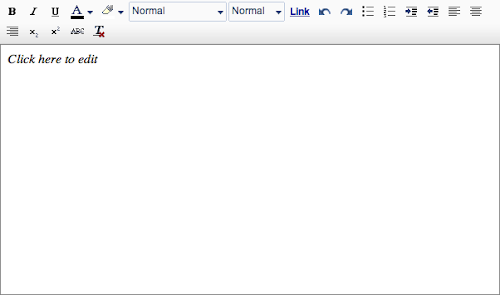
Let us know in the comments if you’ve used or plan to use the Closure Library rich text editor in your project. If you have questions, please post them to the discussion group.
21 comments:
T T said...
July 14, 2010 at 2:07 PM
T T said...
I would most definitely be looking to use this in my projects. My only concern/question is will there be any drawbacks of having italic text defined as "i" tags (Blogger ate my tag) vs defining the styles outside of the markup?
July 14, 2010 at 2:08 PM
Unknown said...
Love the idea of a simpler, more efficient WYSIWYG editor to replace the clunky old editors we've been dragging around for countless years.
What I don't love is my first impression of the HTML that comes out of this thing. Double line break (new paragraph) is denoted with a DIV containing a single BR element? Are you kidding me?
July 14, 2010 at 3:16 PM
Anonymous said...
That's the browser content editable implementation of webkit. Browser makers copied IE's original model which doesn't produce the cleanest HTML. You'll get either p with br or div with br for empty paragraphs.
July 14, 2010 at 5:02 PM
Michal M. said...
Well, it had been there for a while I think. It is not new to closure library.
My biggest concern is how to add pictures to it in a convenient way. Would be great!
July 14, 2010 at 11:45 PM
dchaffin said...
Wow ... I agree with justin ... if that box below the editor on the demo page is supposed to be its HTML output, then thanks, but no thanks. When I changed the color of some text, it actually put a font tag in there with class and color attributes! That and the new div every time you hit enter instead of a new paragraph. Ugh ... this looks like Microsoft HTML.
July 15, 2010 at 7:00 AM
Stefan Matei said...
Seriously? b tags, i tags, br's in div's, and font tags?
No, I don't plan to use it and I don't encourage anyone to unless they're using it for emails or private messages or the likes.
Under no circumstances should you use this to produce code for a live site. Because (among other issues with poorly formatted HTML) you know... there's that big search engine called GOOGLE that's not gonna index your lousy formatted content too well.
July 15, 2010 at 7:14 AM
Steve B said...
Anyone test this with content that is copied and pasted from Microsoft Word? I'd think about switching over if it had good support for MS Word tags removal.
July 15, 2010 at 7:24 AM
Anonymous said...
I am thinking of using this in an ASP.NET CMS called Umbraco. As an alternative to the default editor.
How easy is it to extend this?
July 15, 2010 at 10:42 AM
Anonymous said...
"abstracts the cross-browser inconsistencies and shortcomings "
No it doesn't. The cross-browser inconsistencies and shortcomings are the terrible HTML produced by Rich Text Editors. If this editor could spit out nice, semantic HTML (ideally with easy customisation to add classes & styles) THEN you would have a product worth switching to.
July 15, 2010 at 10:42 AM
DavidJCobb said...
Wow. This editor is just dreadful. Hey guys, try typing centered text and switching back to left. You'll get a left DIV inside the center DIV, instead of the editor just closing the center DIV.
This editor functions EXACTLY as all the other crap editors do: it leverages inconsistent browser functionality.
(I'd like to see someone hack up something more semantic with contentEditable. Tried once, though -- insanely difficult.)
July 15, 2010 at 10:54 AM
Alex said...
This is the worst HTML output I've ever seen from a rich text editor! Get it together Google!
July 15, 2010 at 10:54 AM
Nick B said...
I was really excited when I saw Google releasing a rich text editor. But the HTML is produces makes it totally useless at the moment.
Hopefully though it marks the start of something that will eventually become a good standards compliant rich text editor. I'll look forward to seeing how the project goes.
July 15, 2010 at 11:30 AM
Anonymous said...
I have just came back from being out and about and to test this editor and oh my god the markup it produces is awful !
< font > tags! I'll think I will pass until the markup it produces is much better.
Looks like I wont attempt to replace the TinyMCE editor in Umbraco anytime soon.
Warren :)
July 15, 2010 at 12:04 PM
Bart Calixto said...
FAIL. And btw, didn't you learn anything from facebook? Make inline comments! it's frustrating to comment something on google something and... I HATE FB!
July 15, 2010 at 4:22 PM
Bart Calixto said...
FAIL. And btw, didn't you learn anything from facebook? Make inline comments! it's frustrating to comment something on google something and... I HATE FB!
July 15, 2010 at 4:22 PM
Shripad Krishna said...
I am sorry to disagree with most of you. What you don't realise is that in the pursuit of having a strictly xhtml compliant output you will end up having a very, very large javascript footprint. But still that should not stop anyone from contributing patches to the editor source now that it is open-sourced. Rather than write "hate" comments buck up and contribute.
From "Closure: The Definitive Guide"
"Unfortunately, not all browsers are capable of undoing each other's
styling, so if a user creates some bold text in Internet Explorer, just using the browser's
execCommand would not un-bold it in Firefox."
This can be seen in BasicTextFormatter plugin, where it uses prepareContentsHtml to handle the conversion of < strong > to < b > tags in firefox.
There are many good things about the editor that everyone seems to miss out on. For one, the plugin system. Have you checked out how easy it is to create custom plugins with the editor? Another is the SeamlessField. Yet another, the customization of the editor toolbar and also custom dialogs.
@Justin: If you want br instead of div, check out TagOnEnterHandler.
"GOOGLE that's not gonna index your lousy formatted content too well" I am sorry to say this, but google uses it in most of its products and it is well tested. (Esp blogger.)
July 15, 2010 at 8:19 PM
andy said...
I'm using TinyMCE and it produces perfectly nice valid markup that is usually not too different to the markup I'd write by hand.
This thing is a monstrosity.
July 17, 2010 at 5:36 AM
Anonymous said...
Awesome. I like this closure library. I hope it's possible to implement custom buttons.
I'd like to rig up an image uploader to integrate with it. I could really make use of that.
Steve
May 4, 2011 at 9:38 PM
Shailendra Verma said...
On FAQ and Docs, these people explained every thing but
"how to compress multiple js file into single using command line for closure compiler application -- its not there".
Use below link for this
http://www.netmagazine.com/tutorials/shrink-javascript-files-google-closure-compiler
October 21, 2011 at 6:51 AM
Jojo P. said...
I have read most of them and learned a lot from them. You are doing some great work!
kamagra
February 20, 2012 at 5:34 AM
Post a Comment